Tailwind CSS Starter
sh
npx create-solito-app@latest my-solito-app -t with-tailwind
sh
npx create-solito-app@latest my-solito-app -t with-tailwind
And just like that, you now have an Expo + Next.js app styled with Tailwind CSS.
You can see the monorepo and what comes installed here.
⚡️ Instantly clone & deploy
Rather than using the CLI, you can click this button to clone the repo and deploy it to Vercel:
🔦 About
This monorepo is a starter for an Expo + Next.js app using NativeWind for its styling & Solito for navigation.
🎨 Customize your theme
Open packages/app/design/tailwind/theme.js
to edit your theme on both Web & Native.
👓 How it works
Fast on every platform
NativeWind lets you use Tailwind CSS while minimizing runtime work on every platform.
iOS and Android
Most approaches to using Tailwind in React Native do something like this at runtime:
ts
const styles = props.className.split(' ').map((className) => makeStyle(className))return <View style={styles} />
ts
const styles = props.className.split(' ').map((className) => makeStyle(className))return <View style={styles} />
This means that every component ends up parsing strings to construct predictable style objects.
NativeWind takes a new approach by turning className
strings into StyleSheet.create
objects at build time with a Babel plugin.
With all that said, the Babel plugin will get used on iOS/Android only...
Web
NativeWind uses Next.js' PostCSS
feature to output CSS StyleSheets.
Yes, that's right. On Web, you're using actual Tailwind CSS.
We aren't parsing className
strings into objects for React Native Web to use. Instead, we're actually forwarding CSS classnames to the DOM. That means you can get responsive styles, dark mode support, & pseudo-selectors with server-side rendering support.
Until recently, this was a pipedream with React Native, but with the release of React Native Web 0.18, it's finally possible.
As a result, using NativeWind with React Native Web doesn't have significant overhead compared to plain old Tailwind CSS in a React website.
If you're planning on making a website with Tailwind, why not use Solito with NativeWind?
You might accidentally make a great native app along the way.
Bringing it together
Components are written using the styled()
higher-order component.
In your app's design system, you can start by building your own UI primitives:
tsx
// packages/app/design/typographyimport { Text } from 'react-native'import { styled } from 'nativewind'export const P = styled(Text, 'text-base text-black my-4')
tsx
// packages/app/design/typographyimport { Text } from 'react-native'import { styled } from 'nativewind'export const P = styled(Text, 'text-base text-black my-4')
Notice that you can set base styles using the second argument of styled
.
You can then use the className
prop, just like regular Tailwind CSS:
tsx
<P className="dark:text-white">Solito + NativeWind</P>
tsx
<P className="dark:text-white">Solito + NativeWind</P>
Take a look at the packages/app/design
folder to see how components are created with ease.
If you're reading the NativeWind docs, you might find that you can use
className
directly without usingstyled
. Since this requires the Babel plugin for all platforms, it won't work with Solito. Be sure to always wrap your components withstyled
.
📦 Included packages
solito
for cross-platform navigationmoti
for animationsnativewind
for theming/design (you can bring your own, too)- Expo SDK 49
- Next.js 13
- Expo Router 2
🗂 Folder layout
apps
entry points for each appexpo
app
you'll be creating files inside ofapps/expo/app
to use file system routing on iOS and Android.
next
packages
shared packages across appsapp
you'll be importing most files fromapp/
features
(don't use ascreens
folder. organize by feature.)provider
(all the providers that wrap the app, and some no-ops for Web.)design
your app's design system. organize this as you please.typography
(components for all the different text styles)layout
(components for layouts)
You can add other folders inside of packages/
if you know what you're doing and have a good reason to.
🏁 Start the app
Install dependencies:
yarn
Next.js local dev:
yarn web
- Runs
yarn next
- Runs
Expo local dev:
yarn native
- Runs
expo start
- Runs
🆕 Add new dependencies
Pure JS dependencies
If you're installing a JavaScript-only dependency that will be used across platforms, install it in packages/app
:
sh
cd packages/appyarn add date-fnscd ../..yarn
sh
cd packages/appyarn add date-fnscd ../..yarn
Native dependencies
If you're installing a library with any native code, you must install it in apps/expo
:
sh
cd apps/expoyarn add react-native-reanimatedcd ../..yarn
sh
cd apps/expoyarn add react-native-reanimatedcd ../..yarn
You can also install the native library inside of packages/app
if you want to get autoimport for that package inside of the app
folder. However, you need to be careful and install the exact same version in both packages. If the versions mismatch at all, you'll potentially get terrible bugs. This is a classic monorepo issue. I use lerna-update-wizard
to help with this (you don't need to use Lerna to use that lib).
🎙 About the creator
Fernando Rojo
Follow Fernando Rojo, creator of solito
, on Twitter: @FernandoTheRojo
Mark Lawlor
Follow Mark Lawlor, creator of NativeWind
, on Twitter: @mark__lawlor
🧐 Why use Expo + Next.js?
See my talk about this topic at Next.js Conf 2021:
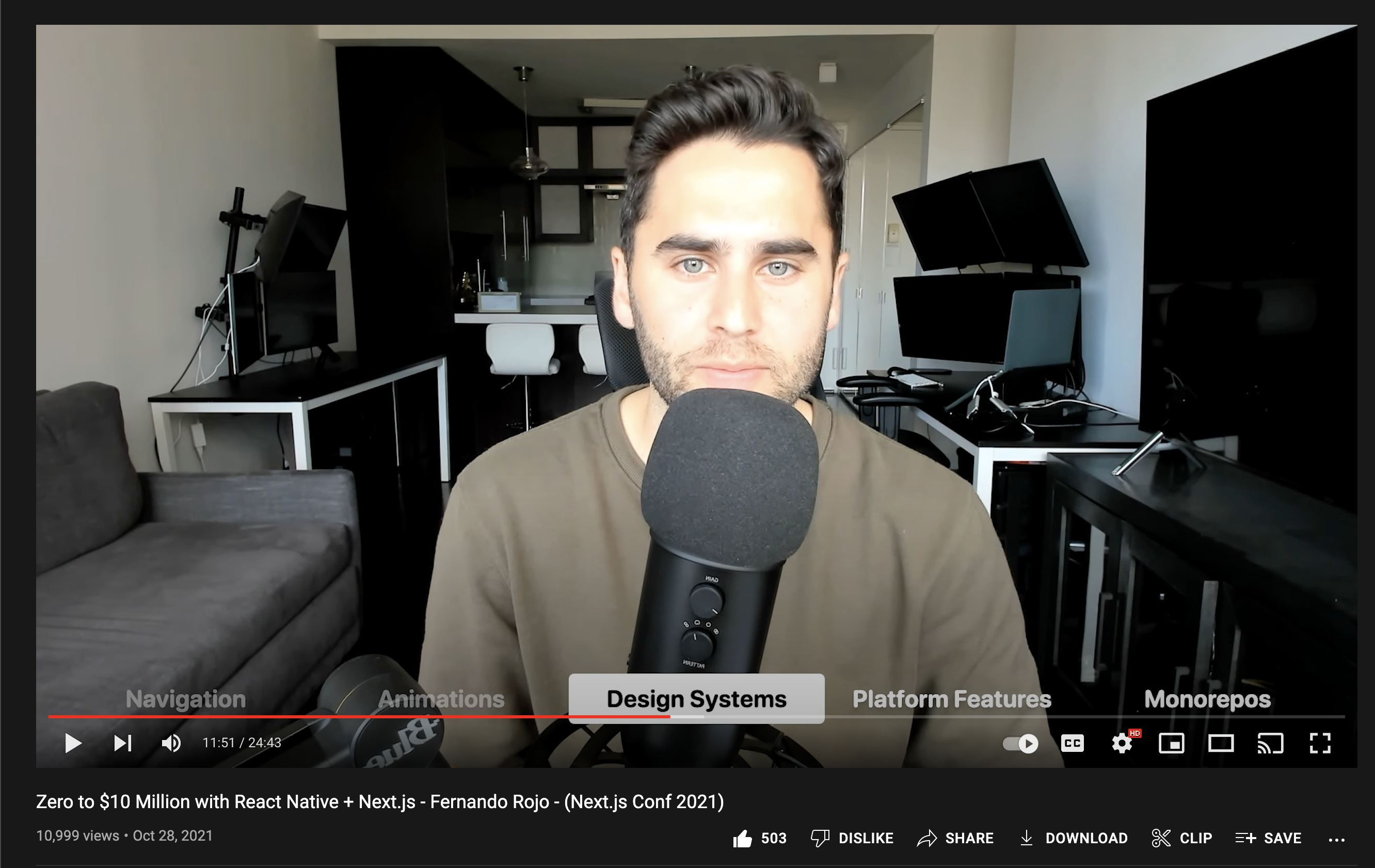